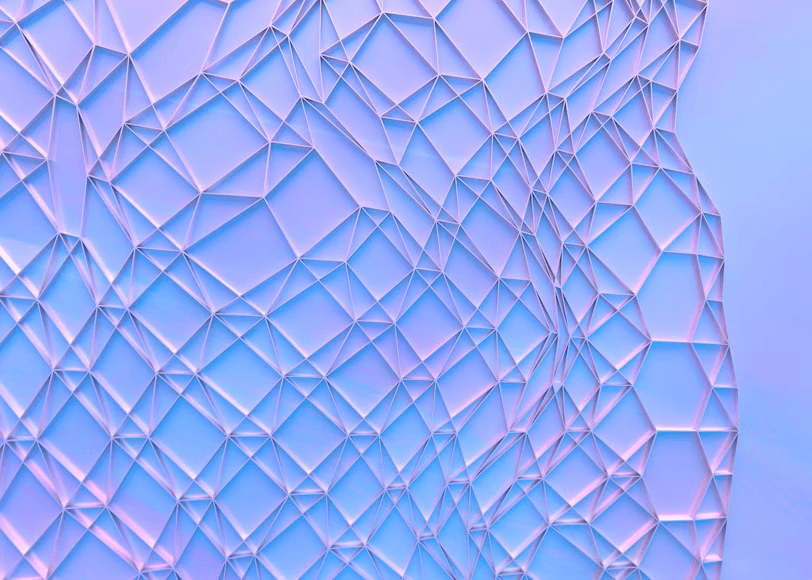
JavaScript Performance Optimization Techniques
Why Performance Matters
Performance is a critical aspect of web development that directly impacts user experience. Slow websites lead to higher bounce rates and lower conversion rates. In this article, we'll explore techniques to optimize your JavaScript code.
Common Performance Bottlenecks
- Excessive DOM manipulation
- Inefficient loops and data structures
- Memory leaks
- Blocking the main thread
- Unoptimized asset loading
Optimization Techniques
1. Use Efficient Selectors
// Inefficient
document.querySelectorAll('.item');
// More efficient
document.getElementById('list').children;
2. Debounce and Throttle
// Debounce example
function debounce(func, wait) {
let timeout;
return function(...args) {
clearTimeout(timeout);
timeout = setTimeout(() => func.apply(this, args), wait);
};
}
// Usage
const debouncedResize = debounce(() => {
// Handle resize
}, 250);
window.addEventListener('resize', debouncedResize);
3. Use Web Workers for Heavy Computation
Web Workers allow you to run JavaScript in background threads, keeping your UI responsive even during intensive operations.
4. Virtualize Long Lists
When displaying large lists, only render the items that are currently visible in the viewport.
Measuring Performance
Always measure before and after optimization using tools like:
- Chrome DevTools Performance panel
- Lighthouse
- Web Vitals